Expression evaluator
Calculate the result of an expression with integers, decimals, numeric variables, string variables for string comparisons, arithmetic and Boolean operators, or a set of functions.
Properties
Property | Description |
---|---|
Expression | The expression to be calculated. The expression can contain an unlimited number of correctly opened and closed parentheses. If the expression does not follow the correct syntax, the converter does not perform the calculation. |
Mode | The direction of the dynamic link:
|
Source
One or more variables identified by:
- {placeholderindex_number}
- Progressive integer equal to or greater than zero. For example,{1}
- {#placeholderplaceholder_identifier}
- String prefixed with#. For example,{#speed}
TIP:
Use any combination of placeholders in any order. Use
any number of placeholders in the expression.
Literals
- Boolean.
- Integers.
- Decimals where the decimal separator must be a point.
- Hexadecimal numbers with the prefix0x.
- Text such as a string delimited by double quotation marks.TIP: Double quotation marks repeated twice ("") are interpreted as an escape sequence.
- Date and time. For example,2017-06-28T15:26:06.0790910.
Operators
Category | Operator | Associativity | Comments |
---|---|---|---|
Unary | Unary minus: - Bitwise NOT: ~ Cast operator: (type) | Right to left | The cast operator (type) applies to all numeric data types and Boolean only. |
Multiplicative | * ,/ , % | Left to right | |
Additive | + , - | Left to right | |
Shift | << , >> | Left to right | Shift and Relational operators apply to these data types:
|
Relational | < , <= , > , >= | Left to right | |
Equality | == , != | Left to right | Equality operators apply to these data types:
|
Bitwise AND, XOR, OR | & , ^ , | | Left to right | |
Logical AND | && | Left to right | |
Logical OR | || | Left to right |
Data types
Data type conventions in the expression evaluator:
- Literal integers are Int32.
- Decimal literals are Double.
- Numerical values are considered to be Int32.
- Literals true and false are interpreted as Boolean.
- The / operator always returns a Double value.
- The % operator generates an exception when there is division by modulo zero.
- The % operator accepts Float and Double operands.
- All functions return a Double value except for sign, which returns an Int32 value.
The
type
of unary cast operators can be of these data types:
- bool, Boolean
- sbyte, SByte
- short, Int16
- int, Int32
- long, Int64
- byte, Byte
- ushort, UInt16
- uint, UInt32
- ulong, UInt64
- float, Float
- double, Double
Operators applied to specific data types:
Operator | Operand1 | Operand2 | Description |
---|---|---|---|
Plus | DateTime | Integral number | Operand2 is interpreted as milliseconds. |
Plus | Integral number | DateTime | Operand1 is interpreted as milliseconds. |
Plus | DateTime | TimeZone (Struct) | Operand2 is interpreted as minutes (TimeZone.Offset). |
Plus | TimeZone (Struct) | DateTime | Operand1 is interpreted as minutes (TimeZone.Offset). |
Plus | TimeZone | Integral number | Operand1 is interpreted as minutes (TimeZone.Offset).Operand2 is interpreted as minutes. |
Plus | Integral number | TimeZone | Operand1 is interpreted as minutes.Operand2 is interpreted as minutes (TimeZone.Offset). |
Minus | DateTime | Integral number | Operand2 is interpreted as milliseconds. |
Minus | DateTime | TimeZone (Struct) | Operand2 is interpreted as minutes (TimeZone.Offset). |
Minus | TimeZone | Integral number | Operand1 is interpreted as minutes (TimeZone.Offset).Operand2 is interpreted as minutes. |
Functions
Functions for use in the expression evaluator:
Function | Syntax | Description | Return data type |
---|---|---|---|
max | max( value1 , value2 , value3 , ...) | Returns the greatest value. | Double |
min | min( value1 , value2 , value3 , ...) | Returns the lowest value. | Double |
avg | avg( value1 , value2 , value3 , ...) | Returns the average of the given values. | Double |
abs | abs( value ) | Returns the absolute value of the given number. | Double |
trunc | trunc( value ) | Returns the integer part of a decimal number. | Double |
ceil | ceil( value ) | Returns the approximated value by excess. | Double |
floor | floor( value ) | Returns the approximated value by defect. | Double |
round | round( value ) | Returns the approximation to the nearest integer. | Double |
sqrt | sqrt( value ) | Returns the square root of the given number. | Double |
sign | sign( value ) | Checks if the given floating point number is negative. Returns 0 if true or 1 if false. | Int32 |
like | like( string_value , pattern ) | Returns true if the string_value parameter matches the pattern . | Boolean |
isempty | isempty( variable ) | Checks if a given NodeID, String, LocalizedText, or DataTime is empty. | Boolean |
if | if( condition , value_if_true , value_if_false ) | Checks if the value_if_true and value_if_false parameters are of the same data type. | value_if_true data type |
left_of | left_of(string, pattern) | Returns the substring on the left of the first occurrence of the regular expression pattern.
IMPORTANT:
Do not use wildcards in patterns.
| String |
right_of | right_of(string, pattern) | Returns the substring on the right of the first occurrence of the regular expression pattern.
IMPORTANT:
Do not use wildcards in patterns.
| String |
Output
The resulting value of the expression.
Example
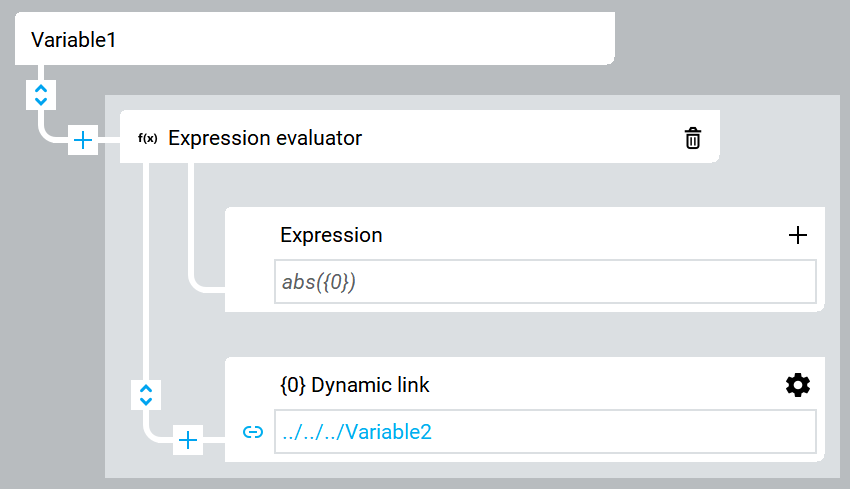
Provide Feedback